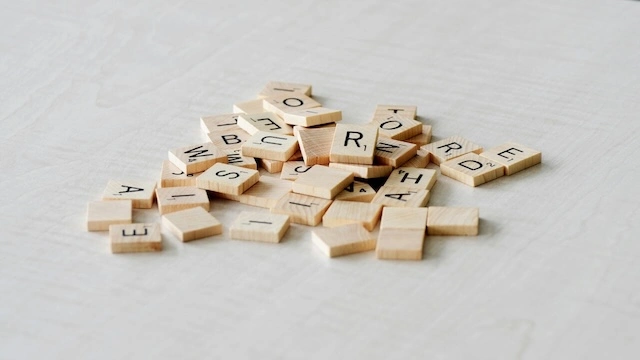
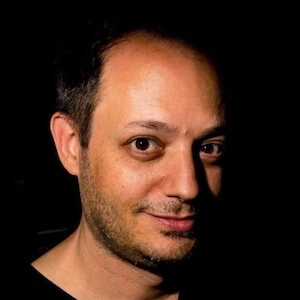
ESLint - Sorting
The sort order of imports, props, object keys, etc. is entirely subjective and even your own opinion about the best order in a particular context is subject to change over time.
Enforcing alphanumeric sorting will keep your code organized and easier to read for everyone on your team.
Alphanumeric
Alphanumeric is the most objective order. A-Z is an order that English speakers learn from childhood, and numeric is universal. It’s independent from programming. It’s automatic. Without thinking, you know that G comes after B, R comes before Y, and 3 comes before 8.
By removing subjectivity from sorting, and sorting things alphanumerically, you make it easy for everyone (including yourself) to quickly find what they’re looking for when reading code.
Non-English alphabet native speakers
Alphabet sorting is English-oriented, but since programming is primarily done in English, it has a positive side-effect of reinforcing the English alphabet for ESL speakers who use a different primary alphabet.
Sorting Rules
There is a great ESLint sorting plugin that supports fix-on-save. It’s called Perfectionist. I recommend using it with the natural
rule configuration. I customize two rules (see below).
plugins: [
// ... other plugins
'perfectionist',
],
extends: [
// ... other extends
'plugin:perfectionist/recommended-natural-legacy',
],
sort-imports
This is my recommended setting. It puts React
at the top, pairs type
imports with their groups, and puts styles
at the bottom for easy access.
'perfectionist/sort-imports': [
'error',
{
type: 'natural',
internalPattern: ['~/**'],
newlinesBetween: 'never',
groups: [
'react',
['external-type', 'external'],
['builtin-type', 'builtin'],
['internal-type', 'internal'],
['parent-type', 'parent'],
['sibling-type', 'sibling'],
['index-type', 'index'],
'side-effect',
['object', 'unknown'],
'style',
],
customGroups: {
value: {
react: ['react', 'react-*'],
},
type: {
react: ['react', 'react-*'],
},
},
},
],
sort-jsx-props
I recommend this modification to sort the reserved props key
and ref
before all other props.
'perfectionist/sort-jsx-props': [
'error',
{
type: 'natural',
groups: ['reserved'],
customGroups: {
reserved: ['key', 'ref'],
},
},
],
Other Sorting
I wrote this this ESLint plugin to sort React hooks dependency arrays.
npm i -D eslint-plugin-sort-react-dependency-arrays
It sorts dependency arrays in useEffect
, useCallback
, etc. This rule keeps dependency arrays consistent with all the other sorting rules.
'sort-react-dependency-arrays/sort': 'error',
Next article: ESLint - Situational rules
Previous article: ESLint - Foundation
Main article: Take your workflow to the next level with ESLint fix on save