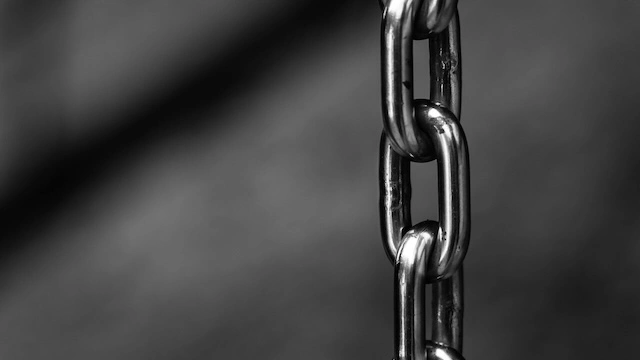
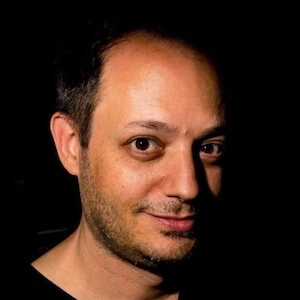
ESLint - Opinionated rules
These are rules which I did not include in the Foundation because they’re more opinionated. I’ll explain why I use them. Feel free to use them or not.
Prohibit enums in TypeScript
The typescript-enum plugin prevents you from using enums. There are good reasons to not use them anymore. The README explains the reasons why.
{
plugins: ["typescript-enum"],
extends: ["plugin:typescript-enum/recommended"],
}
Enforce naming conventions
The check-file plugin provides automatic enforcement of the naming conventions you use in your projects. The README explains how to use it. The rules are subjective and based on how your company likes to name things.
Having rules to automatically enforce your naming conventions saves time, especially for people who are new to your project, and avoids having to bring it up in code reviews.
Only use arrow functions
React is built upon functional programming principles. Functional code is best written using arrow functions. Using arrow functions for everything adds consistency to your code.
Adding the prefer-arrow plugin to your ruleset will enforce this.
Here are some additional reasons why I only use arrow functions:
- They’re already used in many places, including closures and anonymous function parameters in functions such as map, filter, and reduce.
- They do not have self-referential scope
this
. You should never usethis
in functional programming or React. - They do not hoist. Hoisting is “magic”. Being explicit about function order adds clarity to your code. If an arrow function is being called, you know it’s always above the line you’re looking at.
- They are shorter and easier to read and write.
- They have implicit returns.
This is the setting I use:
'prefer-arrow/prefer-arrow-functions': [
'error',
{
classPropertiesAllowed: false,
disallowPrototype: true,
singleReturnOnly: false,
},
],
You will need to disable this rule for *.d.ts
files in your ESLint config overrides
.
{
files: ['**/*.d.ts'],
rules: {
'prefer-arrow/prefer-arrow-functions': 'off',
},
},
Prefer if else over switch
There are numerous articles written about how using switch
is less ideal than if else
, and should probably not be used at all. This plugin prevents switch
from being used in your code base. It does not support “fix”, however, some IDEs have the ability to convert them. It shouldn’t take long to replace all your switch statements after you install and enable the no-switch-statements plugin.
Previous article: ESLint - Situational rules
Main article: Take your workflow to the next level with ESLint fix on save