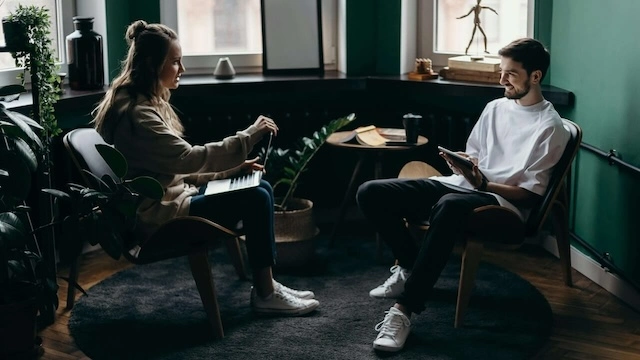
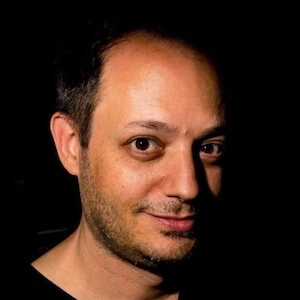
Empathetic Coding
Have you ever inherited a component which is difficult to use?
Problems such as:
- It doesn’t accept props you’d naturally expect, such as
className
. - It accepts
className
, but classes don’t work unless you use!important
, or maybe your class doesn’t get applied at all. - Due to complexity or other reasons outside your control, refactoring is not an option.
- You have to hack a solution around the limitations of the component to get your task done, and by doing so, you create technical debt.
Soft skills are often mentioned in contrast to programming skills. However, soft skills can also be applied to the “hard skills” of programming.
The way to avoid or minimize these types of issues and build components that others appreciate using is by following a philosophy I call “empathetic coding”.
tl;dr
- There are many coding philosophies, but most are based on engineering solutions, while empathetic coding is based on solutions focused around your fellow developers.
- When writing components, you’re choosing between adding conveniences or limitations. Empathetic coding puts the focus on convenience first.
- Empathetic coding makes your components more flexible and easier to use.
Common Philosophies
On your path to becoming a proficient developer, you likely have encountered coding philosophies like KISS, DRY, YAGNI, etc.
You may have heard sayings such as:
premature optimization is the root of all evil
and
make it work, make it right, make it fast
In theory, applying these philosophies can help you write clean, concise code today, and make it easier to change and maintain in the future. In practice, sometimes applying these philosophies can result in over-engineering because they’re advocating engineering solutions.
What is important to remember is that a person in the future will need to understand and use your components (including future you), potentially without you being available to answer questions. They may need to use your components in ways you cannot predict today.
Convenience vs Limitations
When building a component, you aren’t writing code just to satisfy the functionality requirements, you’re also making a series of decisions about how developers will use your component. These choices are mostly tradeoffs between convenience and limitations.
An empathetic approach prioritizes convenience over limitations. Limitations reduce flexibility and therefore should be considered carefully. By adopting this mindset, you are more likely to only add limitations which are necessary.
As a result, your components will be more flexible and will make other developers lives easier.
Convenience-First Mindset
A big benefit to the convenience-first mindset is that it encourages you to keep components simpler. This also makes them easier to test and maintain.
It’s sometimes easy to get caught in a trap of trying to predict every possible variation of functionality, and you can end up over-engineering a component.
When you make your components convenient to use, it makes them easy to compose, and developers can extend or customize the functionality without having to modify the underlying component code.
Real World Examples
I will be releasing a series of articles demonstrating what a convenience-first mindset looks like in practice. Each article will show a real world component, built step-by-step, with each decision explained.
These components are built in React and styled with Tailwind, but the ideas can be applied to general development and whichever styling library you prefer.